Challenge #1: Ball Passing
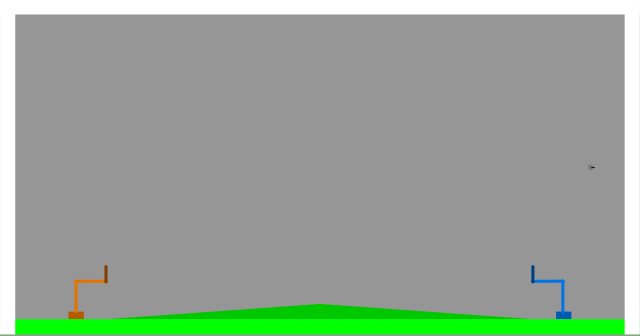
You get points for touching the ball
with the hand of the same color as the ball.
You lose points for touching anything else,
or for the ball touching anything else.
There is a small additional cost to the
torque you apply (so it's better to be relaxed).
Your AI has to play without your help.
However, it does NOT have to run in real time.
This is a cooperative challenge, so submissions
must include source and a description of the approach.
Please give credit when including others' ideas and code.
The challenge will be considered won once we can
maintain a net-positive score, but
it will remain open indefinitely to improvements
and alternate approaches.
The current environment code is available on the main page
Or use "svn checkout http://invivo.googlecode.com/svn/trunk/ invivo"
(Or if you prefer C# and .NET, try this instead:
"svn checkout http://invivo.googlecode.com/svn/extras/dotNet invivo")
It's in python, and you'll need to get
pygame and pybox2d installed to make it work.
(You'll also need python-ming if you
want to generate flash animations.)
There is a very simple JSON network interface
if you wish to implement your solution
in some other language.
Email me if you plan to participate and/or
want an invite to the project's mailing list.
-Simon (simonfunk@gmail.com)
[Back to Index]
This is the interface to each bot:
|
|
|
|
Interface01 is the i/o interface to a three-jointed bot
with one touch sensor and a global sound sensor.
Any position variable is an (x, y) tuple of floats representing a location,
which may be in world or local coordinates as specified. All positions
are in the same units.
Position-related velocities are also (x, y) tuples. Angular velocities are scalars.
Measurements (such as angles) are taken at the end of the most recent time step.
Events (such as sounds) are accumulated during the timestep (exact time is not available).
Static/Configuration info:
.position = where the base of the robot is located in world coordinates.
.numjoints = 3 (order is base, elbow, hand)
.cost = score cost per second of (torque/torquemax)^2 (per each joint)
.torquesmax[n] = maxium abs torque joint n can exert.
Sensors:
.angles[n] = current angle of joint n.
.angvel[n] = current angular velocity of joint n.
.handpos = position of the center of the hand relative to .position
.touch = list of (position in hand coords, magnitude, reward) for any touch sensations on the hand.
.ballpos = position of the ball in world coordinates.
.ballvel = velocity of the ball.
.ballrot = angular velocity of the ball.
.ballcol = "color" of ball, from -1 (prefers left) to 1 (prefers right)
.sound = list of (worldpos, magnitude, reward) for any sound events
Controls:
.torques[n] = current torque exerted by joint n.
|
|
|
|
|
Here is a sample controller (the one shown
in the above animation). This is the ONLY
file you should change:
|
|
|
|
import math
class Controller(object):
"Test controller for Interface01."
def __init__(self, interfaces):
"Interfaces is a pair of Interface01 objects."
self.bots = interfaces
def update(self, time):
self.foo(self.bots[0], time)
self.foo(self.bots[1], time*1.234 + 10.)
#self.bar(self.bots[1])
def bar(self, bot):
for i in range(3):
bot.torques[i] = -bot.angles[i] * 3. - bot.angvel[i] * .01
def foo(self, bot, time):
bot.torques[0] = math.sin(time ) * bot.torquesmax[0]
bot.torques[1] = math.sin(time*1.7) * bot.torquesmax[1]
bot.torques[2] = math.sin(time*2.3) * bot.torquesmax[2]
|
|
|
|
|
[Back to Index]
|